Nailing your first job as an Android developer — Part 1: Build your portfolio
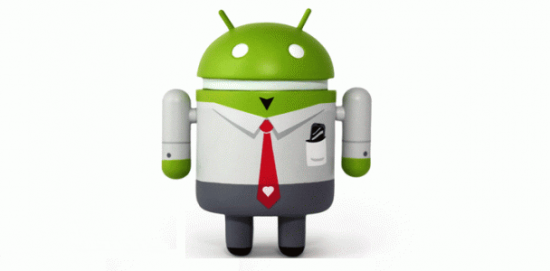
This is part of a multi-part series about how to get your first job as an Android developer. This part focuses on the first step, developing your knowledge and create a portfolio that will attract recruiters
The second part will focus on how to apply to job offers and adapt your resume and cover letter:
The third and last part will explain the different types of interviews you might go through and how to get ready for them.
N.B.: I am neither a coach nor a recruiter but I have spent the last few months hunting for my dream jobs, helped by an awesome coach, and recently nailed it. These posts are a summary of what I’ve learned during the process and would like to share with others. I am open to feedback and suggestions!
If you have already built a few apps and are familiar with dependency injection, data persistence, design patterns etc… You can go through this part quickly and jump to part 2. I’ll recommend reading the part “Why a GitHub profile?” and “Write contents” anyways.
If you are just getting into Android development this will give you a good road map on what to learn and in which order. If you are not familiar with the technology and concept I am talking about don’t worry I have added links toward resources that will help become a good android developer.
Build Apps
Before even talking to you, companies want to see what type of projects you’ve worked on and what you are able to do. It is important to build a nice portfolio with a couple of apps.
Java or Kotlin?
You should include at least one project in Java and one in Kotlin.
Until not so long ago all the apps were developed in Java and most companies still have legacy code in Java. It is still really important to be able to read and write basic Java. But nowadays most of the new development are made in Kotlin and if you don’t know it you will have way less opportunities
If you don’t know any of the languages I’ll recommend starting with Java then learn Kotlin. Both languages run on the JVM and are pretty similar so making the switch to any of them will be easy. Kotlin is just so much more enjoyable that if you start with it, it will be really hard to find the motivation to learn Java.
For more comparisons between Java and Kotlin:
If you already know Java and want a good introduction to Kotlin
To improve your Kotlin knowledge:
Which pattern?
The MVC (Model View Controller) pattern is great to build simple apps and discover Android development but you will run into a lot of problems if you build bigger applications with it. As soon as you understand what is an Activity, a Fragment, a Layout etc… move away from it and go explore other patterns.
Most companies I’ve talked to use the MVVM (Model View ViewModel) pattern so it is essential to build at least one app with it. But the MVP (Model View Presenter) pattern is easier to get your head around and I have been asked several times during interviews the difference between both so I’ll recommend to have a look at it first and maybe build an app with it.
For more differences between each of them and know which one you should use:
MVC:
MVP:
MVVM:
If you want to be a bit fancy you can also look into the MVI (Model View Intent) pattern. I haven’t came across any company that use it but it is always a good thing to show that you have experimented with the different patterns
More information about MVI:
Once you are quite familiar with the patterns and understand how they are organized you should get familiar with the clean architecture principles and use repositories and dependency injection in your apps.
For more information about architecture in Android
Take a look at the android blueprint to have a full example of how architecting your app
The most common library that helps you create a dependency injection pattern is Dagger2 so it is important to know the main principles of it but if you are developing in Kotlin I strongly recommend looking into Koin
What kind of app?
I don’t think any recruiter would expect a junior developer to have created a full app by him/herself with a lot of functionalities. But you have to show them that you have tackled some real life problems and explore some of the options available today.
One of the main problems in Android is data persistence, if the user goes offline for a couple of minutes he/she should still be able to see some contents in your app and have some interactions with it. Build a simple app that pulls a list of images from an API like Unsplash and displays them into a recyclerview. When the user clicks on one of the images show another screen with more details about the picture. Copy those data into a local database like Room to avoid downloading the same data twice. Make sure your app is testable and that the API source could be changed really easily.
Experiment with RxJava, Coroutines, Flow, WorkManager etc…
Test your app with unit tests (go try JUnit5) and UI tests (with Espresso).
You don’t have to know everything, technology and API will change overtime. But show the recruiter that you are curious and aware of today’s problems and trends.
Find examples and inspiration by going through the gGoogle Codelabs
If you are looking for more ideas on apps to develop go take a look at this training. Courses are free but if you want the certifications and someone to look at your apps you have to pay:
Here’s 30 cool android libraries and projects you could use or get inspired by:
Why a GitHub profile?
Having an app on the play store is the best but as a junior developer you might not have a fully developed app to show and that’s ok. You can point the recruiters toward a link where the source code is located. The best way to do it is to have your projects on GitHub as public repositories so they are accessible to everyone. It also show that you have some basic knowledge of Git and GitHub which is more than essential as a developer to have
For a quick overview of Git:
For a more complete course on Git:
Because most of the recruiters are not developers and also not everyone will take the time to compile your app, for each app make sure to have a nice README.md that includes :
- Screenshots / animated GIFs of the app
- A description of the functionalities
- A list of the main libraries you used
- Language and pattern chosen for the project
Fore more informations on how to build a nice README.md:
You can find some example on my GitHub profile for the projects that are done:
LinkedIn Profile
Why?
LinkedIn is massively used by the recruiters today, some might come straight to you through LinkedIn so why miss those opportunities? Also almost every time you will apply for a job you will be asked for your LinkedIn profile, it is essential to have one nowadays.
What should it contain?
Your LinkedIn profile is a detailed resume with all your work experiences, diploma, certifications, volunteer experience, skills etc… the more the better.
For each work experience and diploma add a detailed description of what you’ve done and, if you can, a link where we can see the projects you’ve worked on.
How to be notified by recruiters?
Set your headline with the job title you are looking for and specify that you are actively looking for new opportunities. Example: “Android Developer available for remote jobs”, “Android Engineer looking for new challenges”…Set your profile to “open to job opportunities” for a better chance to be contacted.
Add connections, find people you went to school with, your teachers, you can also add developers that are working for the companies you would like to work at. Have them endorse your skills and write short recommendations to prove that you have some basic knowledge in the field.
For more details explanation on how to build your profile:
My LinkedIn profile as an example (I am open to feedback on it in the comment section):
Write contents
Today companies are looking for people with strong communication skills. You have to be able to communicate with your peers when you have a problem, ask questions, explain a concept to another developer who might not be familiar with it etc…
Share your knowledge about a technical (or not technical) subject by writing a blog post about it, show not only that you are able to explain a concept clearly to other people but also that you are willing to share your knowledge with others, two soft skills that really interest companies.
What to write about?
It might not seem easy to find a subject as a junior developer but trust me there are a tons of subjects you can write about.
While developing your apps you will run into problems for which you can’t find a straight or satisfying answer online. Technology and API change quickly and yesterday’s solutions might not be adaptable to today’s problems. You might find a part of the answer you are looking for in one source and another part of the answer in another source. Compile them into one blog post.
If you are worried about not being competent enough, don’t forget that to master a subject you have to teach it first.
How to write a blog post?
Keep it simple and straightforward. A simple and concise example is better than long paragraphs.
Add code to demo what you say by creating Gist and/or a public GitHub repo with the full example.
More about how and why to start writing here:
If this seems like a lot, it is normal, building a good portfolio and strong knowledge takes time. You don’t have to be an expert into everything I mentioned but you have to be aware of it. The main goal is to show recruiters that you are curious and know how to stay up to date with the technology.
Thanks for reading! If you are a developer or a recruiter let me know what you think in the comments!
Next part will be about how to apply to job offers and adapt your resume and cover letter: