Creating multiple constructors for Data classes in Kotlin
Migrating from Java to Kotlin — War story 1
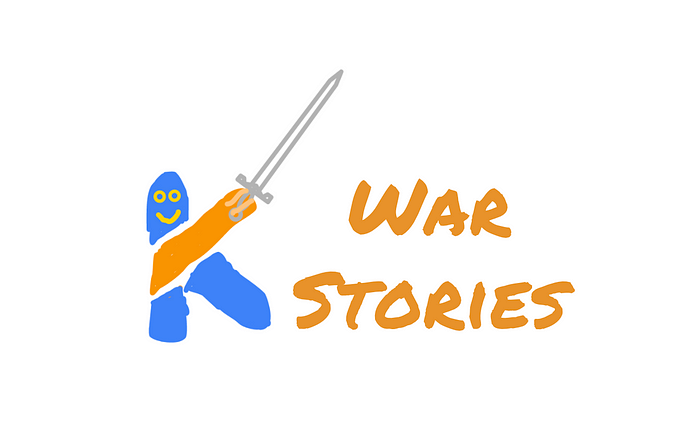
It’s been a month since I joined Annyce in migrating our codebase away from Java to Kotlin. Every time she sent a PR, the amount of code she added was always remarkably lower then the amount she had taken away while still accomplishing the tasks at hand.
EVERY. SINGLE. TIME.
I started out with all our data classes so that I could get the hang of working with Kotlin, that’s after I personally went through the Kotlin Koans and read Kotlin for Android Developers by Antonio Leiva. I later moved on to migrating more complex stuff before finally graduating to writing Kotlin from scratch.
I’ll attempt to highlight all the areas that stood out to me during this period. It’s obvious but worth mentioning again that your codebase wasn’t built in a day so it’ll definitely take some time to achieve a 100% Kotlin codebase.

Creating multiple constructors for Data classes in Kotlin
aka Secondary constructors with multiple parameters
Data classes in Kotlin are immutable and it’s easy enough to create a constructor for a data class with multiple fields. Note that it’s compulsory to have a primary constructor in a data class. It’s also compulsory to have the val or var keyword before the variable name, which you can get away with in normal classes and secondary constructors.
data class Person(val name: String, val age: Int)
What if you need several constructors in the same data class? You can use Secondary constructors to achieve this. According to the documentation,
“If the class has a primary constructor, each secondary constructor needs to delegate to the primary constructor, either directly or indirectly through another secondary constructor(s). Delegation to another constructor of the same class is done using the this keyword”
data class Person(val name: String) {
constructor(name: String, age: Int) : this(name)
}
Another option is the @JvmOverloads annotation which generates multiple constructors based on the arguments in the constructor. Again, according to the documentation, @JvmOverloads
“Instructs the Kotlin compiler to generate overloads for this function that substitute default parameter values.
If a method has N parameters and M of which have default values, M overloads are generated: the first one takes N-1 parameters (all but the last one that takes a default value), the second takes N-2 parameters, and so on.”
data class Person @JvmOverloads constructor(val name: String,
val age: Int? = 0)
Going by the excerpt above, the only overloaded constructor generated in this case has one parameter — val name.
Note that when you include annotations in your class declaration, you have to explicitly write out the constructor keyword just before the parenthesis. This is a lot more concise than the first option and it works as intended.
Thanks to Michael Obi and Segun Famisa for reviewing.
Thought this was great? Please don’t forget to “Recommend” and “Share”.