Coroutines for Android
Coroutines are a great way to handle asynchronous programming where it also offers an immense easy way to switch between context from foreground to background and vice-versa.
Android activities or fragments come with their own lifecycles and also to achieve structured concurrency, running coroutines with GlobalScope will not be a good option so one of the better approaches is to have a coroutine scope limited with the Android component.
If you’re using Android Architecture Components, that makes it even easier to use coroutines.
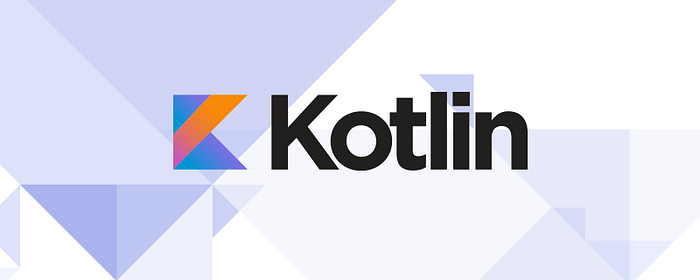
Let’s see the first approach where coroutines are used with Android Architecture Components.
Coroutines with ViewModel
1. Create a job object
2. Create coroutine scope object with job and the context on which coroutine should run
3. On that coroutine scope object use any of the coroutine builders like launch or async
4. Override onCleared method and cancel job
class UserViewModel : ViewModel() { private val job = Job() private val uiScope = CoroutineScope(Dispatchers.Main + job) fun doSomeOperation() {
uiScope.launch {
//Working on UI thread
print(Thread.currentThread().name)
//Use dispatcher to switch between context
val deferred = async(Dispatchers.Default) {
//Working on background thread
10 + 10
}
//Working on UI thread
print(deferred.await())
}
} override fun onCleared() {
super.onCleared() job.cancel()
}
}
Now let’s see the second approach where coroutines are used in an activity or a fragment without android architecture component.
Coroutines with Activity/Fragment
1. Implement CoroutineScope
2. Initialize job object
3. Override CoroutineContext object and assign with job + on which thread coroutines should work using Dispatchers
4. Cancel the job in onDestroy method
class MainActivity : AppCompatActivity(), CoroutineScope {
private lateinit var mJob: Job
override val coroutineContext: CoroutineContext
get() = mJob + Dispatchers.Main
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
mJob = Job()
launch {
//Working on UI thread
print(Thread.currentThread().name)
//Use dispatcher to switch between context
val deferred = async(Dispatchers.Default) {
//Working on background thread
10 + 10
}
//Working on UI thread
print(deferred.await())
}
}
override fun onDestroy() {
super.onDestroy()
mJob.cancel()
}
}
So far so good now what about exceptions, in case of exception raised within launch coroutine builder it will make the app to crash, it’s like an unhandled exception whereas in case exception raised inside async it should be handled by the programmer.
Let’s see how to handle the exception, the simplest approach is to create a CoroutineExceptionHandler object and pass it to coroutine builder. What happens is that whenever any exception is raised inside coroutine builder it will auto handle it.
launch(handler) {
//Working on UI thread
print(Thread.currentThread().name)
//Use dispatcher to switch between context
val deferred = async(Dispatchers.Default) {
//Working on background thread
throw IOException()
}
//Working on UI thread, exception will not be raised util await is called.
print(deferred.await())
}private val handler = CoroutineExceptionHandler { coroutineContext, throwable ->
Log.e("Exception", ":" + throwable)
}
Note: CoroutineExceptionHandler is invoked only on exceptions which are not expected to be handled by the user, so registering it in async builder has no effect.
Coroutines can be synchronous also
There is often a time when synchronous executions are required and coroutines can handle those also by defining the coroutine start method with async coroutine builder.
launch(handler) {
val job1 = async(Dispatchers.Default, CoroutineStart.LAZY) {
//Working on background thread
10
}
val job2 = async(Dispatchers.Default, CoroutineStart.LAZY) {
//Working on background thread
20
}
//Working on UI thread
Log.v("DATA", "Output"+job1.await() + job2.await())
}
what happens is that coroutine will not start its execution until the await method is called. This kind of usage is useful when coroutine execution should start based on some event.
This kind of execution takes time as until first coroutine execution is done the second coroutine will not start. To fix this the simple approach is to call job.start() method before job.await() it will ensure coroutines to run in parallel.
Coroutines with callbacks
There may be a situation where the results are not available immediately, it may come through callbacks. To handle this kind of situation there is a coroutine builder for that and it’s suspendCoroutine
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
mJob = Job()
launch(handler) {
val result = getFromCallback()
Log.v("Res",""+result)
}
}private val handler = CoroutineExceptionHandler { coroutineContext, throwable ->
Log.e("EXC", ":" + throwable)
}private suspend fun getFromCallback() = suspendCoroutine<Int> {
Handler().postDelayed(object : Runnable {
override fun run() {
//Return result directly
it.resume(15)
//or, return result which is wrapped by Result success
it.resumeWith(Result.success(15))
//or, return result which is wrapped by Result failure
it.resumeWith(Result.failure(AssertionError()))
}
}, 2000)
}
Gradle dependencies for coroutines
dependencies {
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-core:1.1.1'
implementation 'org.jetbrains.kotlinx:kotlinx-coroutines-android:1.1.1'
}
Rules for Proguard or R8
If you are using coroutines than don’t forget to add these rules to the Proguard file.
# ServiceLoader support
-keepnames class kotlinx.coroutines.internal.MainDispatcherFactory {}
-keepnames class kotlinx.coroutines.CoroutineExceptionHandler {}
# Most of volatile fields are updated with AFU and should not be mangled
-keepclassmembernames class kotlinx.** {
volatile <fields>;
}
Coroutines are really easy to use although it does require a bit extra care over exceptions, what is needed when coroutines child is canceled and a proper context switch. Hope this article will help to add coroutines to your projects!
Following is the link where I have shown how android runtime ktx can help to get started with coroutines
https://youtu.be/hegQjuULLUQ