Better dependency injection for Android
Simplifying dependency injection with Kotlin and Koin — (examples shown here are in Koin 0.2.x) — Check the latest changes of Koin
Since I began to work as a developer, I’ve seen a bunch of building technologies: from JBoss and other JEE stuffs, to light apps with Spring framework and even more concise frameworks like Giuce (for my GWT needs). On Android, the #1 solution that I find (almost) everywhere is Dagger.
What I’ve learned: stay away from your assembling tech as possible and use constructor injection. Decoupling your components means also decoupling from your glue tech. That’s why the @inject annotation has been created. Below, a good refresh of the Martin Fowler words about DI (dependency injection) pattern:
In every DI solution you will find the same concepts: instances container, reference binding, module definitions, graph dependency… The injection of components is generally done by introspection (scan your class to look for attributes to be injected), which can be costly on mobile devices. That’s why solution such as Dagger emerged with the idea of processing everything and generating source code injection plumbing for you. But I think Kotlin can allow smarter solution design.
Another way with Kotlin?
On Android development platform, alternatively to Dagger, you can see interesting initiative like Toothpick that avoid the need of generated code (but use introspection). Keep in mind that all of these solutions has been made in the Java language context. We could make it better with Kotlin ;)
Sebastien Deleuze proposed some months ago, a functional way of declaring dependencies into Spring Framework thanks to Kotlin:
The gain is quiet clear. No need of proxy, introspection or code generation. Just declare your components, assemble it and reuse it thanks to lambda expressions, functions and DSL. All the plumbing behind is in the end highly simplified, and its global overhead too.
Writing a new project whereas we already have Kotlin projects such as Kodein? My idea is to be compact and straight forward, in order to ensure the main value: allow you to describe your app context in a few lines and reuse it easily everywhere!
The functional DSL approach consists of a simple declaration of your content within lambdas:

The Module class brings the main DSL for your components definition. You can now declare your dependencies in the context() method, within the declareContext function:
- provide — allows to provide a component in a functional way
- get — retrieves your component
Note that all your bean definitions are lazily declared. An instance will be made only when an injection is called. We resolve dependencies from context directly with the get() function, which makes bean resolution for you (no need to specify typing, Kotlin is strong enough).
Now just build your module context and inject your components from it. That’s all!
Insert Koin and start injecting
Koin is an open source project to help you write your dependency injection in few lines.
Let’s build an Android app with koin-android module (complete sample app wiki & sources). Start with an update of your build.gradle:
// repository if needed
allprojects {
repositories {
jcenter()
}
}// Koin for Android
dependencies {
compile 'org.koin:koin-android:0.2.0'
}
Write a module class and describe your dependencies (here we have okhttp, retrofit and a WeatherService class):

The AndroidModule is a Koin module specialized for Android needs: you can get your injections and applicationContext, resources & assets within your dependencies. e.g: I need my server url here.
Extend the KoinContextAware interface in your Android Application class, to help you setup your Koin context:
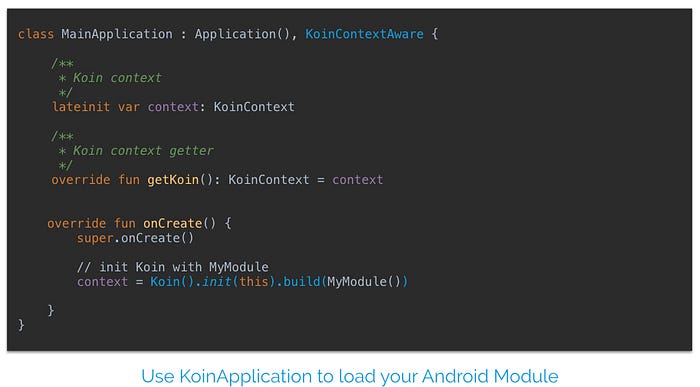
That’s all! You’re ready. Now you can inject the WeatherService class, with by inject() function extension:

That’s it! Dependency injection in just a few lines :) No Proxy, No code generation, No introspection. Just give it a try!

More about Koin
KOIN is a simple dependency injection framework that uses Kotlin and its functional power to get things done!
The Koin project has just been started on github/koin. Check the project to learn more about it. Feedbacks are welcome 👍