A more flexible Checkbox in Material3
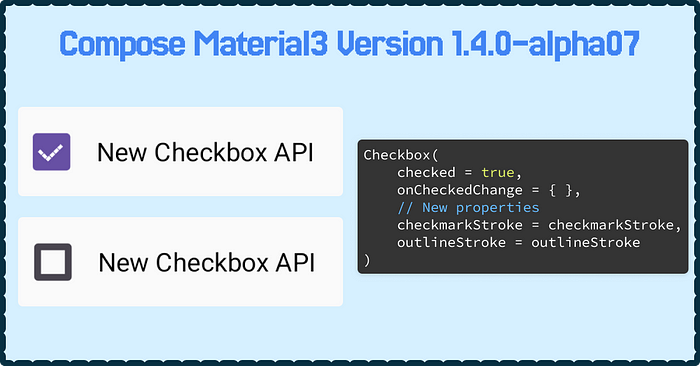
Starting with version 1.4.0-alpha07 of compose.material3:material3
we get a new API for Checkbox, which provides us an option to customize the stroke of the checkmark
and checkbox-outline
.
Existing API
@Preview(showBackground = true)
@Composable
fun CheckboxOldSample() {
val checkedState = remember { mutableStateOf(true) }
Row(
modifier = Modifier.fillMaxWidth(),
verticalAlignment = androidx.compose.ui.Alignment.CenterVertically
) {
Checkbox(
checked = checkedState.value,
onCheckedChange = { checkedState.value = it }
)
Text(text = "Old Checkbox API"
}
}
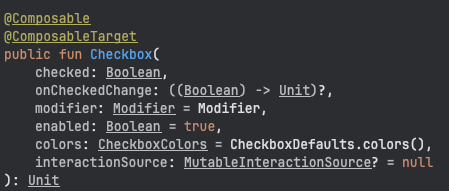
New API implementation
@Composable
fun CheckboxWithRoundedStrokes() {
val strokeWidthPx = with(LocalDensity.current) { floor(CheckboxDefaults.StrokeWidth.toPx()) }
val checkmarkStroke =
remember(strokeWidthPx) {
Stroke(
width = strokeWidthPx,
cap = StrokeCap.Square,
join = StrokeJoin.Round,
pathEffect = PathEffect.dashPathEffect(floatArrayOf(2f, 6f))
)
}
val outlineStroke = remember(strokeWidthPx) {
Stroke(width = 8f)
}
val checkedState = remember { mutableStateOf(true) }
Row(
modifier = Modifier.fillMaxWidth(),
verticalAlignment = androidx.compose.ui.Alignment.CenterVertically
) {
Checkbox(
checked = true,
onCheckedChange = { },
// New properties
checkmarkStroke = checkmarkStroke,
outlineStroke = outlineStroke
)
Text(text = "New Checkbox API")
}
}
Demo
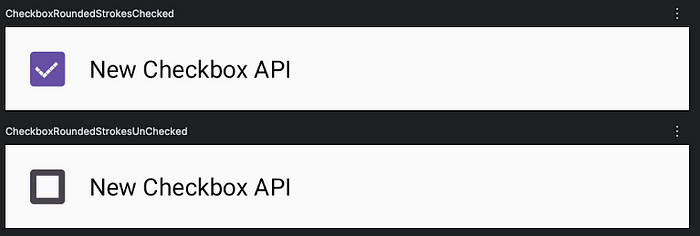