Five Useful Kotlin Extensions you may use
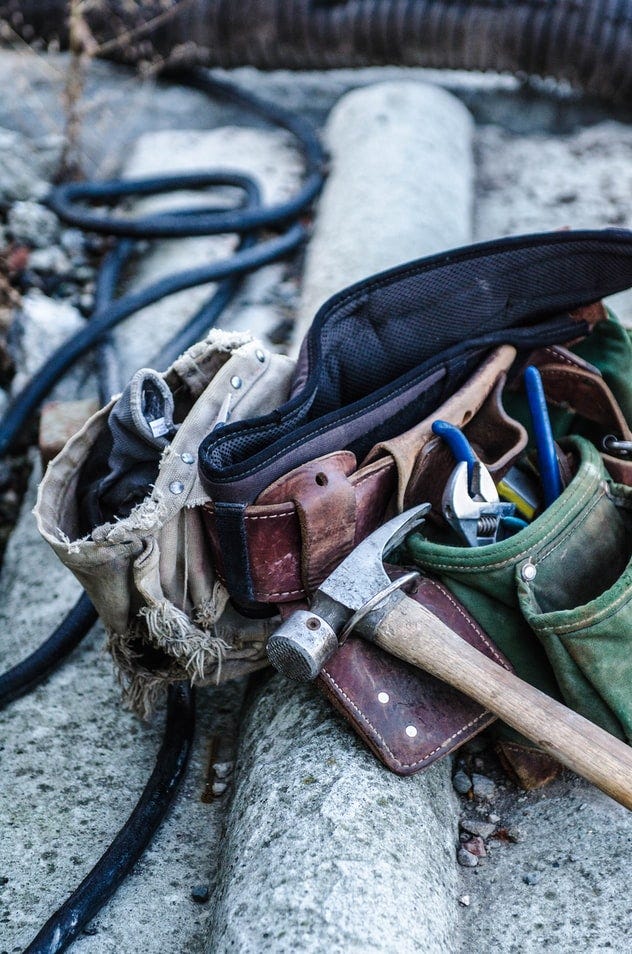
Extensions is one of the coolest features in Kotlin as it provides the ability to extend a class with new functionality without even inheriting that class!
Remember how we were doing that using other programming languages that doesn’t support extensions, by inheriting the class or use design patterns such as Decorator, but what extensions will do is to reduce writing that boilerplate code by defining just a functions, today we will talk about the common extensions in Kotlin.
1- IsNull
In a normal way this how we are checking for the nullability.
if (something == null){}
Let’s make it more legit by using Kotlin extensions.
fun Any?.isNull() = this == null
So our code will be like this, how cool is that!
if (something.isNull()){}
2- Price Amount
Here is an extension for formatting a string to decimal format also, we can overload this extension and use it with Double or Long.
let’s say we have an E-commerce app, and every product has its own price so theses extensions will help us to format the price to a pattern that we want.
so The usage of it will be:
println("11".toPriceAmount())
println("05".toPriceAmount())
println(12.0.toPriceAmount())
And the output:
11.00
5.00
12.00
3- Date Format
What we were doing is creating a utils class for the Date Format, people may argue with me about this but IMHO making an extension for Date Format will make the code cleaner.
So let’s say we have a note-taking app so each note shows a date it was made also, we can convert that date to UnixTime and store it in the database
The usage of it:
val currentTime = System.currentTimeMillis()
println(currentTime.getTimeStamp())
println(currentTime.getYearMonthDay())
println("2020-09-20".getDateUnixTime())
The output:
Sunday, September 20, 2020 - 10:48:26 AM
2020-09-20
1600549200000
4- ObjectSerializer
Actually this is one of my favorite extensions because it shows the power of Kotlin extensions which is to make an extension for an interface, yes any class implements that interface can use this extension.
Let’s say we have a flashcards app, so we need to export the cards to send them to a friend or to restore them later, this ObjectSerializer will help us to serialize the flashcards to String or ByteArray so we can store them in a file and restore them later using deserialize extension.
In the code below we can see that we can serialize every class implements Serializable, it’s not about this case specifically but imagine how this will help us if we applied it on something bigger :)
Usage of it:
val kotlinExtention = "Kotlin Extensions ObjectSerializer"
val serializedString = kotlinExtention.serialize()
println(serializedString)
println(serializedString.deserialize() as String)
The output:
kmonaaafheaaccelgphegmgjgocaefhihegfgohdgjgpgohdcaepgcgkgfgdhefdgfhcgjgbgmgjhkgfhcKotlin Extensions ObjectSerializer
You can find the full version of the file here:
5- Generic Extension Functions
In this one, we will have the power of generic, by creating an extension that is taking a generic type we can apply it for every class extends or implements that generic.
This one is very useful when we want to edit to a third-party library or an API so instead of inheriting that class, we can make use of extensions which will help us to reduce that boilerplate code.
So If we have an app and we need to search for a name of something using this extension to set list of names to AutoCompleteTextView Adapter, or even if we need to copy the name list after choosing a RandomNames so this will help us to get the Name list and store the in a clipboard .
In the example below I have created a Student class that extends User, and our extension is printing a list of names.
Usage of this one:
The output:
[Kotlin, Alex, Mohammed]
So this is what we have for this article, thank you for reading and I hope you enjoyed it and will use some of them in your apps.
Stay tuned for the next Article which is will be about Android Kotlin Extensions.